안녕하세요. 오늘은 기존에 작성한 네이버 뉴스 클로링 코드에서 첫 번째 코드의 자세한 크롤링 과정을 포스팅 하겠습니다.
새롭게 변경된 첫 번째 코드의 자세한 크롤링 과정입니다!
네이버 뉴스 크롤링 전체 코드를 확인하고 싶으신 분들은 아래 링크를 확인해주세요!
[Crawling] 네이버 뉴스 크롤링 코드 변경
안녕하세요! 네이버 뉴스가 24년 1월 25일부터 페이지가 보여주는 방식이 변경되면서, 이전 포스팅에서 진행했던 첫 번째 코드를 사용할 수 없게 되었습니다. 그래서 변경된 페이지에서 적용되는
yhj9855.com
이전에 사용되었던 BeautifulSoup으로 크롤링 했던 코드의 자세한 과정이 궁금하신 분들은 아래 링크를 확인해주세요!
[Crawling] 네이버 뉴스 크롤링 - 2
안녕하세요. 오늘은 기존에 작성한 네이버 뉴스 크롤링 코드에서 첫 번째 코드의 자세한 크롤링 과정을 포스팅 하겠습니다. 네이버 뉴스 크롤링 전체 코드를 확인하고 싶으신 분들은 아래 링크
yhj9855.com
첫 번째 코드는 Python으로 특정 날짜의 네이버 게임/리뷰 카테고리의 기사들을 크롤링하는데, 제목과 링크만 가져오는 코드였습니다.
- 기본 링크 설정하기
크롤링을 시작할 때 크롤링하고자 하는 페이지의 링크를 설정하는 것이 가장 중요합니다.
위의 그림처럼 2025년 01월 07일의 게임/리뷰 카테고리의 뉴스를 크롤링하고자 한다면, 원하는 정보가 담긴 링크는 아래와 같습니다.
https://news.naver.com/breakingnews/section/105/229?date=20250107
해당 링크에서 가장 중요한 것은 date=20250107 부분인데요!
네이버 뉴스의 경우, 날짜를 링크 자체에서 구분할 수 있게 되어 있습니다.
그렇기 때문에 특정 날짜의 기사들을 크롤링하고 싶다면 date 뒤에 연도+월+일을 붙이면 된다는 사실을 알 수 있습니다.
해당 부분을 코드로 변경하면 아래와 같습니다.
# 수정하고자 하는 메인 링크
link = 'https://news.naver.com/breakingnews/section/105/229?date='
# 스크랩 하고 싶은 날짜를 년도월일 나열해준다.
# 날짜를 쉽게 바꾸기 위해 date를 따로 선언해준다.
date = '20250107'
# 메인 링크는 링크에 날짜가 붙은 구조이기 때문에 이렇게 작성해준다.
main_link = link + date
# 기사의 수, 제목, 링크를 받아올 예정이기 때문에 정보를 담아줄 데이터 프레임을 생성한다.
Main_link = pd.DataFrame({'number' : [], 'title' : [], 'link' : []})
[크롤링 하고자 하는 URL 찾기 Tip]
링크를 확인할 때 오늘 날짜, 첫 페이지와 같이 기본적으로 접속하면 바로 볼 수 있는 URL로 확인하는 건 피하시는게 좋습니다.
기본으로 설정된 URL은 해당 URL만 특별하게 설정되어 있거나, 보이는 링크와 실제 링크가 다른 경우도 종종 있기 때문에 오류가 발생하기 쉽습니다.
아래 그림처럼 '오늘' 날짜의 뉴스 기사에는 date 표시가 되어있지 않기 때문에 초기 링크를 설정할 때 어려움이 있을 수 있습니다.

- 기사 더보기 클릭 및 URL 확인하기
원하는 날짜의 모든 기사를 크롤링하기 위해서는 아래 그림처럼 기사 더보기 버튼을 클릭해서 기사를 끝까지 업로드 해야 합니다.
이 때, 중요한 것은 기사를 업로드 해도 URL이 변경되지 않는다는 것입니다!
기사 더보기를 웹 페이지 안의 내용이 달라졌음에도 URL이 달라지지 않기 때문에 이전에 진행했던 정적 크롤링으로는 진행할 수가 없는 것입니다.
이렇게 URL이 변경되지 않고, 데이터가 변경되는 것을 동적 페이지라고 부릅니다.
동적 페이지는 오직 1가지 방법으로만 크롤링이 가능한데요!
바로 Selenium을 활용한 동적 크롤링 입니다.
Selenium을 활용한 동적 크롤링을 진행하기 위해서는 크롬 드라이버 설치가 필수적입니다.
크롬 드라이버 설치가 되어 있지 않거나, 자신의 크롬 버전과 크롬 드라이버 버전이 맞지 않으시는 분들은 아래 링크에 설명된 방법을 통해서 크롬 드라이버를 먼저 설치해주셔야 합니다!
크롬드라이버 설치하기 (최신버전과 다른 크롬드라이버 설치)
안녕하세요!최근에 크롬 드라이버를 설치하는 페이지가 변경되어 새롭게 포스팅을 하겠습니다! 이전 포스팅이 궁금하신 분들은 아래 포스팅을 참고해주시면 됩니다!https://yhj9855.com/entry/%ED%81%AC
yhj9855.com
- 코드로 기사 더보기 버튼 클릭하기
이제 어떤 방향으로 크롤링을 해야하는지 알았기 때문에 기사를 모두 업로드해서 크롤링하는 방법만 남았습니다.
다음으로 고려해야 할 문제점은 저희가 코드로 기사 더보기 버튼을 클릭해야 하는 것과 기사 더보기 버튼의 끝을 알아야 한다는 것입니다.
아래 그림처럼 기사를 기사 더보기 버튼을 지속적으로 클릭하다보면, 더 이상 해당 버튼이 등장하지 않는 것을 보실 수 있습니다.
해당 부분을 보고 저희가 알 수 있는 것은 기사 더보기 버튼을 계속 클릭하다보면, 더 이상 버튼이 없어서 오류가 발생하는 지점이 생긴다는 것입니다!
이제 개발자 도구 창을 열어, 기사 더보기 버튼이 어떤 HTML 구조를 가지고 있는지 확인하는 작업이 필요합니다.
개발자 도구에 대한 자세한 설명은 아래 링크를 확인해주세요!
크롬 개발자 도구 사용하기
안녕하세요. 오늘은 크롤링을 진행하기 위해 반드시 필요한 개발자 도구를 사용하는 법에 대해서 포스팅 하겠습니다. 크롬 개발자 도구란? 크롬 브라우저에 직접 내장된 웹 개발 도구로, 웹 페
yhj9855.com
저희는 기사 더보기 버튼의 HTML 구조를 알고 싶은 것이기 때문에 아래 그림처럼 따라합니다.
①번의 아이콘을 클릭한 후, ②번(=원하는 정보)을 클릭하면 원하는 정보의 HTML 구조로 바로 이동이 됩니다.
원하는 정보의 HTML 구조를 확인해보니, 아래와 같이 나왔습니다.
<a href="#" class="section_more_inner _CONTENT_LIST_LOAD_MORE_BUTTON" data-persistable="false">기사 더보기</a>
[HTML 구조의 뜻]
- 'a' 태그 : 링크가 담겨져 있는 공간이면 해당 태그를 사용합니다. 현재 버튼을 클릭하면 새로운 기사가 등장하기 때문에 해당 태그를 사용했습니다.
- href: 링크의 주소를 가지고 있는 부분입니다. 여기서는 #을 사용하여, 페이지를 변동하지 않겠다는 옵션을 지정해주었습니다.
- class: 태그의 속성을 나타내는 부분입니다. 크롤링을 진행할 때 많이 사용되는 부분으로 ID가 없을 경우에는 이름처럼 사용되기도 합니다.
- data-* : HTML5에서 지원하는 사용자 정의 데이터 속성입니다. 여기서의 옵션은 특정 동작이나 상태를 저장하지 않도록 지시하는 것을 의미합니다.
기사 더보기 버튼의 HTMl 구조를 알았으니, 오류가 발생할 때까지 버튼을 클릭하는 코드를 작성합니다.
service = Service('chromedriver.exe')
driver = webdriver.Chrome(service=service)
driver.get(main_link)
# 웹 페이지 로딩을 기다리는 코드로, 초는 더 짧아도 된다.
time.sleep(3)
# 기사 더보기 버튼
more_button = driver.find_element(By.CLASS_NAME, 'section_more_inner._CONTENT_LIST_LOAD_MORE_BUTTON')
# 기사 더보기가 몇 개가 있을지 모르기 때문에 오류가 날 때까지 누르는 것으로 한다.
# 여기서 발생하는 오류란 버튼을 찾을 수 없다 즉, 버튼이 없을 때 발생하는 오류이다.
while True :
try :
more_button.click()
time.sleep(3)
except :
break
위의 코드에서 보면, time.sleep 코드를 사용하는 것을 보실 수 있습니다.
동적 크롤링을 작성하실 때 생각보다 많이 놓치실 수 있는 부분인데요!
동적 크롤링은 코드로 크롬을 띄우고, 직접 웹 페이지에 들어가서 해당 페이지에 있는 내용을 크롤링하는 작업입니다.
그렇기 때문에 웹 페이지 로딩이 되지 않은 상태에서 접근을 시도하면 오류가 발생하는데, 이 때 발생하는 오류가 특정 HTML을 찾을 수 없다는 오류가 발생합니다.
이로 인해 초보자분들께서는 웹 페이지가 로딩이 되지 못했다고 생각을 못하고, 코드 내 오류가 있다고만 생각을 하여 한참 시간을 소모하는 경우를 많이 봤습니다!
이를 방지하기 위해서 time.sleep 명령어를 웹 페이지가 변경될 때마다 꼭 넣어주셔야 합니다.
몇 초를 기다리는지는 웹 페이지 안의 영상, 이미지 여부나 인터넷 속도에 따라서 다르지만 보통 1~5초 사이 라고 보시면 됩니다.
- 기사의 제목과 링크 가져오기
마지막으로 크롤링 목적인 기사의 제목과 링크를 가져오기만 하면 첫 번째 코드의 크롤링이 완료됩니다!
버튼을 확인했던 것처럼 개발자 도구에서 기사의 제목과 링크가 어떤 HTML 구조를 가지고 있는지 확인해보겠습니다.
HTML 구조를 확인해보니 아래와 같이 나왔습니다.
<a href="https://n.news.naver.com/mnews/article/119/0002911455" class="sa_text_title _NLOG_IMPRESSION" data-clk="alist" data-rank="34" data-gdid="8812A0A8_000000000000000002911455" data-imp-url="https://n.news.naver.com/mnews/article/119/0002911455">
<strong class="sa_text_strong">LCK컵, 15일 개최…우승 팀은 국제 대회 출전권 획득</strong>
</a>
[HTML 구조의 뜻]
- 'strong' 태그 : HTML에서 텍스트를 굵게 보이기 위한 태그입니다.
이전 기사 더보기 버튼의 HTML 구조와 매우 유사한 것을 알 수 있습니다.
저희가 수집해야 할 정보는 아래와 같습니다.
- 기사의 제목 : a태그 속 strong태그 텍스트
- 기사의 링크 : a태그 속 HTML 구조의 href
해당 부분을 코드로 변경하면 아래와 같습니다.
# 기사의 제목과 링크가 모두 담긴 a태그를 모두 찾는다.
articles = driver.find_elements(By.CLASS_NAME, 'sa_text_title._NLOG_IMPRESSION')
# a태그 내 기사의 제목과 링크를 따로 저장한다.
for i in range(len(articles)) :
# 기사의 제목
# strip을 사용하여 눈으로 확인할 수 없는 양 끝의 공백을 제거한다.
title = articles[i].text.strip()
# href 부분을 가져온느 방법
# a태그 내 href를 가져온다.
link = articles[i].get_attribute('href')
# 번호는 0부터 시작하기 때문에 1을 더해준다.
li = [i+1, title, link]
Main_link.loc[i] = li
- 액셀 파일로 저장
이제 크롤링 작업은 완료되었고, 크롤링한 데이터프레임을 엑셀 파일로 저장하도록 하겠습니다.
# 엑셀을 잘 관리하기 위해서 크롤링 날짜를 파일 이름에 포함한다.
excel_name = 'news_' + date + '.xlsx'
with pd.ExcelWriter(excel_name) as writer :
Main_link.to_excel(writer, sheet_name='링크', index=False)
이런 과정을 통해서 특정 날짜의 모든 기사의 제목과 링크를 크롤링하는 코드가 완성되었습니다!!
[전체 코드]
import pandas as pd
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
import time
from selenium.webdriver.common.by import By
from openpyxl import *
link = 'https://news.naver.com/breakingnews/section/105/229?date='
date = '20250107'
main_link = link + date
Main_link = pd.DataFrame({'number' : [], 'title' : [], 'link' : []})
service = Service('chromedriver.exe')
driver = webdriver.Chrome(service=service)
driver.get(main_link)
time.sleep(3)
more_button = driver.find_element(By.CLASS_NAME, 'section_more_inner._CONTENT_LIST_LOAD_MORE_BUTTON')
while True :
try :
more_button.click()
time.sleep(3)
except :
break
articles = driver.find_elements(By.CLASS_NAME, 'sa_text_title._NLOG_IMPRESSION')
for i in range(len(articles)) :
title = articles[i].text.strip()
link = articles[i].get_attribute('href')
li = [i+1, title, link]
Main_link.loc[i] = li
excel_name = 'news_' + date + '.xlsx'
with pd.ExcelWriter(excel_name) as writer :
Main_link.to_excel(writer, sheet_name='링크', index=False)
이전 코드와 마찬가지로 Xpath, CSS_Selector를 사용하지 않았는데, 해당 부분을 사용하면 쉽게 크롤링을 할 수 있지만 HTML 코드가 복잡하거나 크롤링을 배우고 싶은 분들에게는 좋은 방법이 아니라고 생각합니다.
기사의 본문을 크롤링하는 두 번째 코드의 자세한 크롤링 코딩 과정을 확인하고 싶으신 분들은 아래 링크를 확인해주세요!!
https://yhj9855.com/entry/Crawling-%EB%84%A4%EC%9D%B4%EB%B2%84-%EB%89%B4%EC%8A%A4-%ED%81%AC%EB%A1%A4%EB%A7%81-3
[Crawling] 네이버 뉴스 크롤링 - 3
안녕하세요. 오늘은 기존에 작성한 네이버 뉴스 크롤링 코드에서 두 번째 코드의 자세한 크롤링 과정을 포스팅 하겠습니다. 네이버 뉴스 크롤링 전체 코드를 확인하고 싶으신 분들은 아래 링크
yhj9855.com
코드에 대해 궁금한 점이 있으신 분들은 댓글로 남겨주시면, 답변 드리겠습니다.
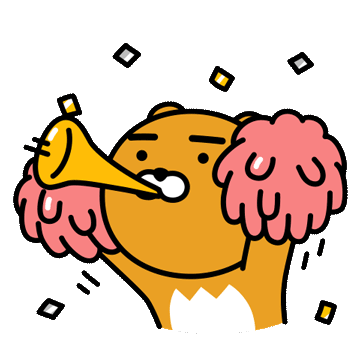
★ 읽어주셔서 감사합니다★
'Python(파이썬) > Crawling(크롤링)' 카테고리의 다른 글
[Crawling] 원신 나무위키 (캐릭터, 성유물) 크롤링 - 1 (51) | 2024.03.31 |
---|---|
[Crawling] 네이버 뉴스 크롤링 코드 변경 (40) | 2024.03.27 |
[Crawling] 네이버 뉴스 크롤링 - 3 (72) | 2024.01.11 |
[Crawling] 네이버 뉴스 크롤링 - 2 (74) | 2024.01.08 |